Grouping validations
Grouping validations are created with static methods:
A grouping Validation
object contains several predicate groups which can be associated with different validatable objects as well as with the same one. Its validity state depends on execution results of all predicate functions in the contained groupes.
Validation.group( Validation(firstName), Validation(lastName) ) .constraint(isAlpha) .constraint(isLongerOrEqual(2)) .constraint(isShorterOrEqual(32))
// firstName, lastName are HTMLInputElement (validatable objects)// isAlpha, isLongerOrEqual(2), isShorterOrEqual(32) are predicate functions
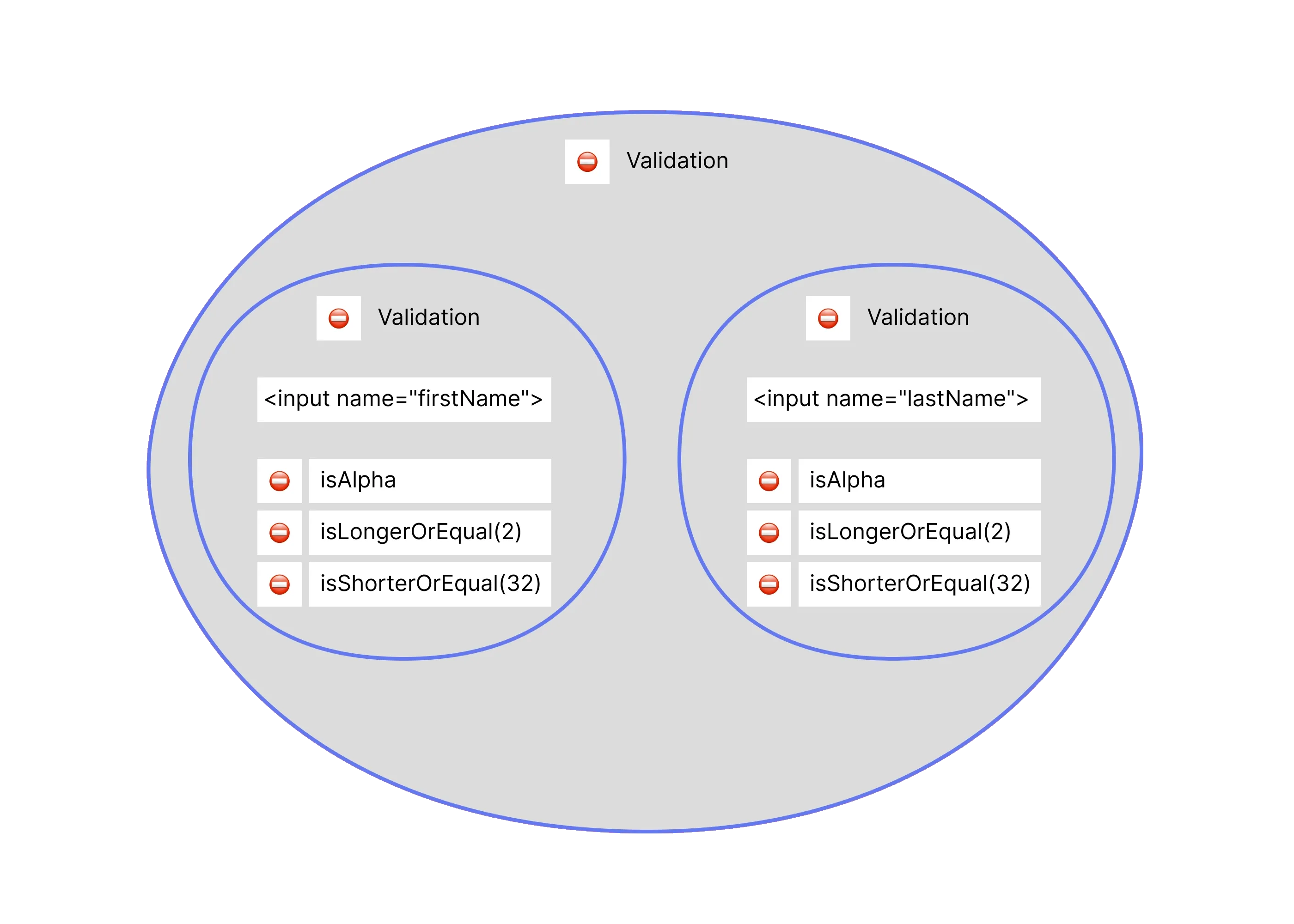
The Validation().constraint()
method invoked on a grouping Validation
adds a predicate function to each predicate group it contains.
Consider this code snippet.
const signupValidation = Validation.group(
Validation.group( Validation(firstName), Validation(lastName) ) .constraint(isAlpha) .constraint(isLongerOrEqual(2)) .constraint(isShorterOrEqual(32)),
Validation.glue(
Validation(password) .constraint(isStrongPassword),
Validation(pwdConfirm),
).constraint(areTwoEqual),);
// firstName, lastName, password, pwdConfirm are HTMLInputElement (validatable objects)// isAlpha, isLongerOrEqual(2), isShorterOrEqual(32), isStrongPassword,// areTwoEqual are predicate functions
Resulting signupValidation
will contain 4 predicate groups which can be accessed through the Validation().constraints
property as signupValidation.constraints
:
-
firstName
:-
isAlpha
-
isLongerOrEqual(2)
-
isShorterOrEqual(32)
-
-
lastName
:-
isAlpha
-
isLongerOrEqual(2)
-
isShorterOrEqual(32)
-
-
password
:-
isStrongPassword
-
areTwoEqual
-
-
pwdConfirm
:areTwoEqual
The Validation
objects that were grouped can be accessed through the Validation().validations
property as signupValidation.validations
.
We can connect UI effects to state changes of the grouping Validation
, its grouped validations, and execution of predicate functions.
// add a UI effect to the grouping validationsignupValidation.changed(toggleAccess(submitBtn));
// add a UI effect to the grouped (nested) validationsconst [ firstAndLastNameV, pwdV ] = signupValidation.validations;
[ ...firstAndLastNameV.validations, pwdV, pwdV ].forEach( (validation, idx) => validation .valid(showStatus(fields[idx])) .invalid(showStatus(fields[idx])));
// add a UI effect to execution of the predicate functions[...signupValidation.constraints].forEach( ([, validator], idx) => validator .valid(showStatus(constraints[idx])) .invalid(showStatus(constraints[idx])));
Now, if we add the grouping Validation
as an event handler it will be running the predicate group associated with the input element passed as the target
property of the Event
object.
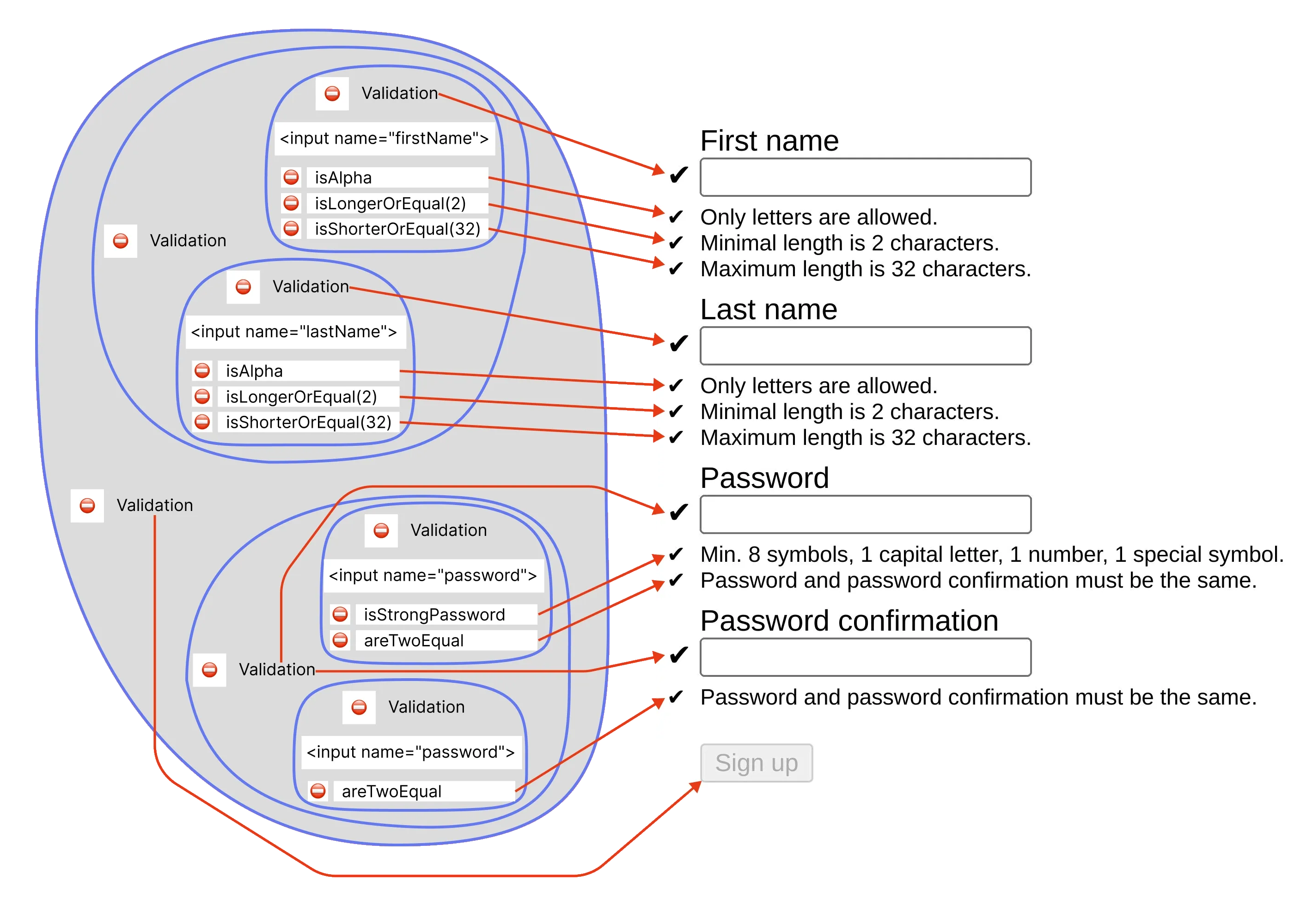
Try it in action in the playground below.